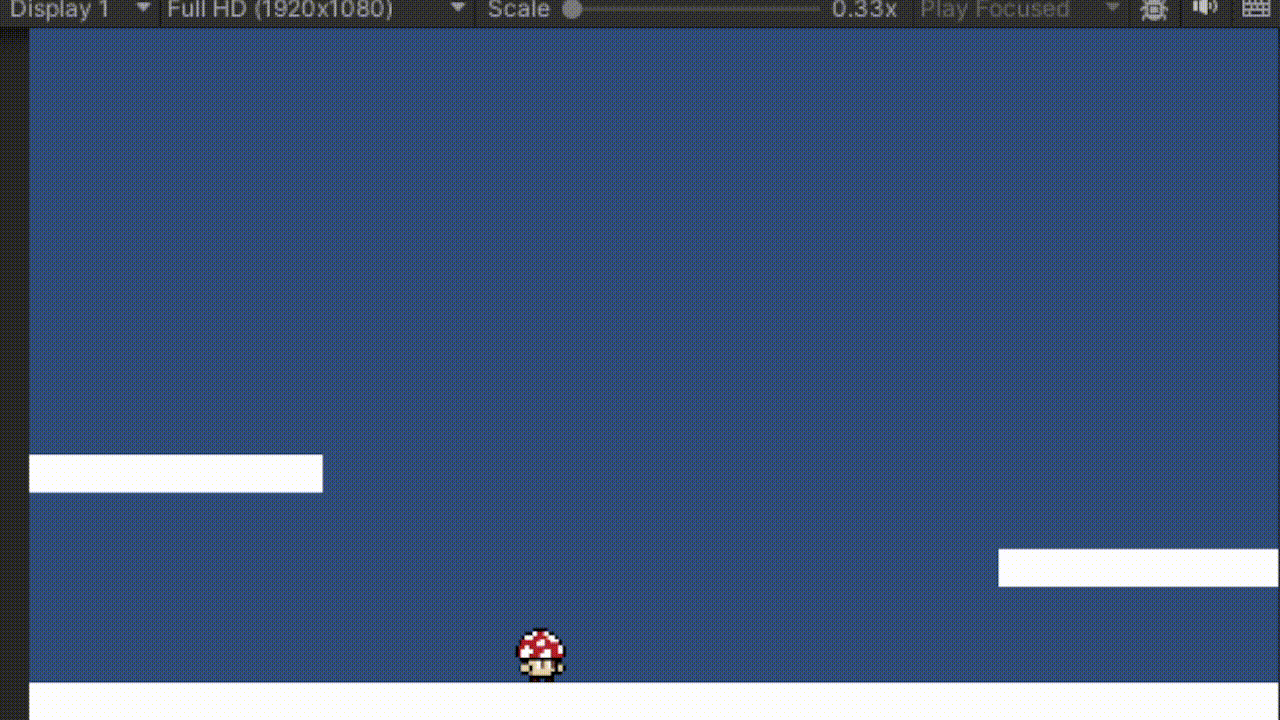
Today, I tested a double jump feature for a 2D platformer controller.
I haven’t had extensive amount of experience with the legacy input system, but I have to say the new input system is confusing at first. Or am I still confused.
At the end, the new input system seems to be the path to take if you want an easy implementation of a variety of hardware controllers users may choose to use.
public void Jump(InputAction.CallbackContext context)
{
// Jump only if grounded
if (context.performed && IsGrounded())
{
rb.velocity = new Vector2(rb.velocity.x, jumpPower);
canDoubleJump = true;
}
// Shorter jump height if jump is tapped
if (context.canceled && rb.velocity.y > 0f)
{
rb.velocity = new Vector2(rb.velocity.x, rb.velocity.y * 0.5f);
}
// Double jump
if (!IsGrounded() && context.performed && canDoubleJump)
{
rb.velocity = new Vector2(rb.velocity.x, jumpPower);
canDoubleJump = false;
}
}